The “Quick Response” commonly known as QR Code has become an essential part of our digital life. Nowadays, almost every smartphone comes with a pre-installed QR code scanner or reader app. QR code gives us easy accessibility for the sharing of a few bits of data in different forms. Here, different forms refer to any kind of text, contact number, identification number, or URL. We can share such data without any actual communication between two devices or one device and paper.
In this tutorial, we will study how to show QR code on an OLED display using Arduino or ESP8266. This tutorial will be useful for Arduino and NodeMCU or ESP8266. If you are new to program ESP8266 or NodeMCU, use this guide to program ESP8266 or NodeMCU using Arduino IDE. If you want to know, how to interface OLED display with NodeMCU, you can check out this post. Now, let’s start with the installation of the required libraries.
Requirements
- Arduino or ESP8266/NodeMCU
- OLED Display (SSD1306 used here)
- Arduino IDE
Installing the necessary libraries for QR code generation on Arduino or ESP
The very first basic step will be installing libraries required for QR Code generation. It again depends on the development board you are using. For the Arduino family, you can use this GitHub library and for ESP8266, NodeMCU or ESP32 you can use this GitHub library.
The best and easiest way to install these libraries is from Arduino IDE’s library manager. You can open library manager from Sketch -> Include Library -> Manage Libraries.
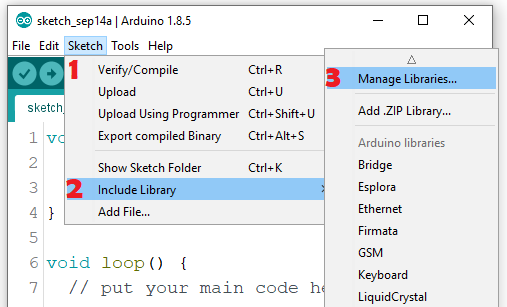
After this, in library manager, search for QR Code, and you will find several libraries for the same!
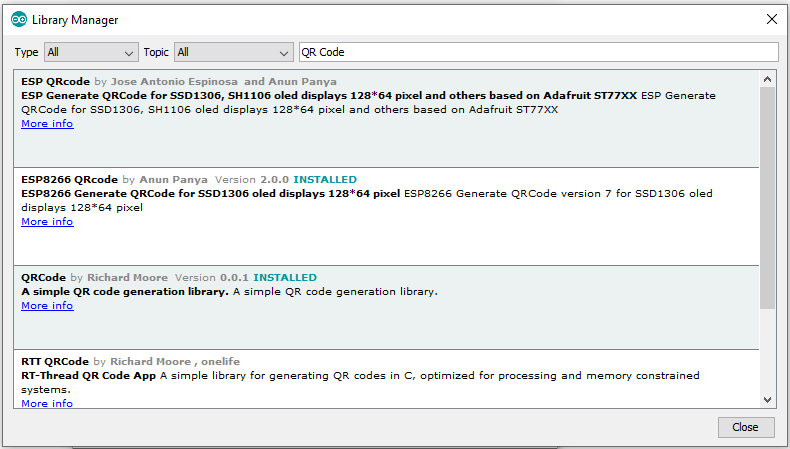
Personally, I will prefer the library among the first two on the list. Both of them are designed for the ESP family. If you are interested to show QR code on OLED using Arduino UNO, choose the board as Arduino and then open library manager. You will get relevant libraries there.
Program to generate and display QR code on OLED
For this tutorial, I will be using library “ESP8266 QRcode” which exposes high level APIs to interact with OLED Display. We just need to some simple function calls to get things working. Before proceeding for coding, we need to include installed libraries.
#include <qrcode.h>
#include <SSD1306.h>
Along with libraries, we also need to define some constants as well.
/*
Address -> depending on OLED display
Pins -> depending on board
*/
SSD1306 display(0x3c, D6, D7);
QRcode qrcode (&display);
The address of the OLED display is totally depending on the display you are using. Also, the pin numbers are depending on the development boards. The full program to show QR code on OLED Display is as below:
#include <qrcode.h>
#include <SSD1306.h>
/*
Address -> depending on OLED display
Pins -> depending on board
*/
SSD1306 display(0x3c, D6, D7);
QRcode qrcode (&display);
void setup() {
Serial.begin(115200);
Serial.println("");
Serial.println("Starting");
//initializing display
display.init();
display.clear();
display.display();
//initializing QR code
qrcode.init();
// create qrcode
qrcode.create("https://iotforgeeks.com");
}
void loop() { }
Thank you
but its give a error.
sketch_mar04a:9:1: error: ‘QRcode’ does not name a type
QRcode qrcode (&display);
^
/tmp/arduino_modified_sketch_10386/sketch_mar04a.ino: In function ‘void setup()’:
sketch_mar04a:24:5: error: ‘qrcode’ was not declared in this scope
qrcode.init();
Hello Neha,
Have you included the QR code library?
ya i have the same error. and i follow your tutorial. I upload my sketch with arduino IDE and my modul use is ESP 32 with pin SDA SCL is 22 23. Please helpme